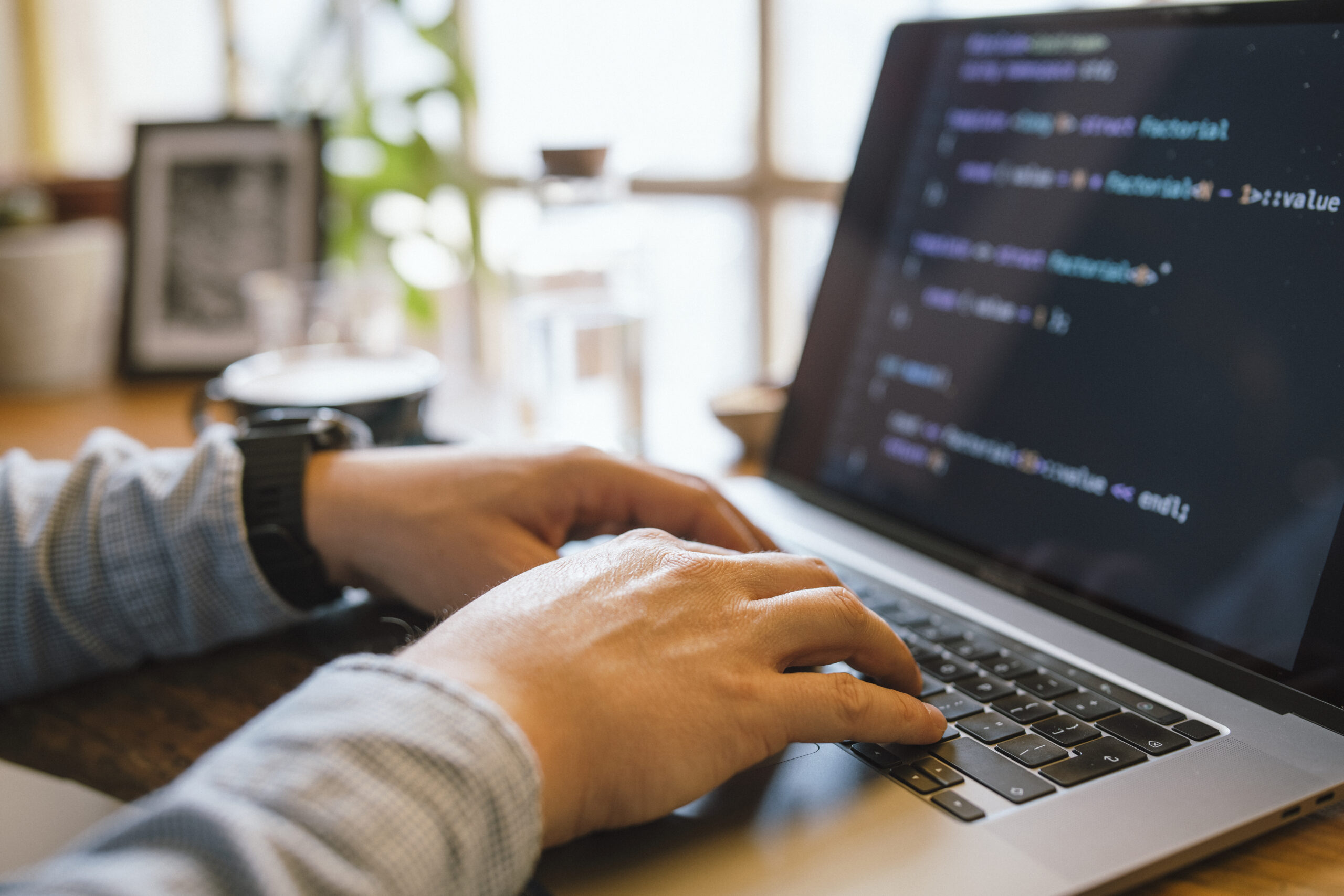
Debugging is Just about the most vital — yet frequently disregarded — techniques in a very developer’s toolkit. It isn't really nearly repairing broken code; it’s about comprehension how and why points go Completely wrong, and Discovering to Feel methodically to resolve difficulties effectively. No matter whether you're a novice or simply a seasoned developer, sharpening your debugging competencies can help you save several hours of annoyance and considerably transform your productivity. Here are several procedures that will help builders degree up their debugging video game by me, Gustavo Woltmann.
Grasp Your Equipment
One of many quickest means builders can elevate their debugging capabilities is by mastering the instruments they use daily. Whilst writing code is a person part of development, realizing how you can connect with it proficiently for the duration of execution is equally vital. Modern progress environments arrive Geared up with highly effective debugging capabilities — but lots of builders only scratch the surface area of what these tools can perform.
Consider, such as, an Integrated Development Atmosphere (IDE) like Visible Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, stage by means of code line by line, and even modify code about the fly. When utilized effectively, they let you notice exactly how your code behaves during execution, that's invaluable for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-stop builders. They permit you to inspect the DOM, watch network requests, check out serious-time efficiency metrics, and debug JavaScript in the browser. Mastering the console, sources, and community tabs can turn aggravating UI challenges into manageable jobs.
For backend or system-degree builders, applications like GDB (GNU Debugger), Valgrind, or LLDB provide deep Management around managing procedures and memory administration. Studying these equipment could possibly have a steeper learning curve but pays off when debugging efficiency challenges, memory leaks, or segmentation faults.
Beyond your IDE or debugger, grow to be at ease with Variation control methods like Git to be familiar with code history, discover the exact second bugs have been launched, and isolate problematic improvements.
In the end, mastering your resources signifies heading outside of default options and shortcuts — it’s about producing an personal knowledge of your improvement surroundings in order that when troubles occur, you’re not dropped at nighttime. The greater you are aware of your applications, the greater time you may shell out fixing the particular challenge in lieu of fumbling by the method.
Reproduce the trouble
Just about the most vital — and sometimes disregarded — measures in efficient debugging is reproducing the issue. Before leaping to the code or building guesses, developers require to create a dependable setting or situation exactly where the bug reliably appears. Without the need of reproducibility, repairing a bug turns into a sport of prospect, generally resulting in wasted time and fragile code changes.
Step one in reproducing an issue is accumulating as much context as possible. Check with thoughts like: What actions led to The problem? Which atmosphere was it in — enhancement, staging, or creation? Are there any logs, screenshots, or error messages? The greater depth you have got, the less complicated it gets to be to isolate the precise circumstances less than which the bug occurs.
When you’ve gathered sufficient facts, attempt to recreate the condition in your local natural environment. This might mean inputting precisely the same data, simulating related person interactions, or mimicking program states. If The difficulty appears intermittently, look at writing automatic checks that replicate the edge situations or point out transitions included. These tests not merely assistance expose the issue and also prevent regressions Later on.
From time to time, the issue can be environment-certain — it would materialize only on particular working systems, browsers, or below distinct configurations. Applying tools like virtual machines, containerization (e.g., Docker), or cross-browser screening platforms might be instrumental in replicating this kind of bugs.
Reproducing the challenge isn’t merely a move — it’s a mindset. It demands persistence, observation, plus a methodical approach. But when you can constantly recreate the bug, you are previously midway to repairing it. That has a reproducible circumstance, You should use your debugging resources a lot more efficiently, exam opportunity fixes properly, and connect far more Plainly using your crew or end users. It turns an abstract complaint into a concrete obstacle — and that’s where builders prosper.
Examine and Fully grasp the Error Messages
Error messages tend to be the most useful clues a developer has when one thing goes Improper. Instead of seeing them as frustrating interruptions, builders need to find out to deal with error messages as immediate communications with the technique. They usually tell you what precisely took place, wherever it occurred, and occasionally even why it transpired — if you understand how to interpret them.
Commence by reading the information very carefully and in complete. Lots of developers, especially when underneath time stress, look at the primary line and quickly begin earning assumptions. But deeper in the mistake stack or logs may lie the genuine root result in. Don’t just copy and paste mistake messages into engines like google — study and fully grasp them very first.
Crack the error down into sections. Could it be a syntax mistake, a runtime exception, or even a logic mistake? Does it place to a specific file and line variety? What module or function brought on it? These concerns can tutorial your investigation and stage you towards the liable code.
It’s also beneficial to be familiar with the terminology from the programming language or framework you’re working with. Error messages in languages like Python, JavaScript, or Java generally follow predictable designs, and Discovering to recognize these can substantially increase your debugging procedure.
Some faults are vague or generic, and in All those cases, it’s essential to look at the context wherein the error transpired. Test related log entries, input values, and recent improvements during the codebase.
Don’t overlook compiler or linter warnings either. These typically precede bigger concerns and supply hints about probable bugs.
Finally, error messages aren't your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint concerns more rapidly, lower debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is one of the most strong instruments in a very developer’s debugging toolkit. When applied correctly, it offers authentic-time insights into how an software behaves, serving to you fully grasp what’s happening underneath the hood without having to pause execution or phase throughout the code line by line.
An excellent logging method begins with understanding what to log and at what level. Frequent logging amounts consist of DEBUG, INFO, Alert, Mistake, and Deadly. Use DEBUG for in depth diagnostic info throughout advancement, Information for general situations (like prosperous start out-ups), WARN for possible challenges that don’t crack the applying, ERROR for actual complications, and Deadly once the system can’t go on.
Keep away from flooding your logs with extreme or irrelevant info. An excessive amount of logging can obscure vital messages and slow down your method. Deal with essential occasions, point out alterations, input/output values, and critical final decision points in the code.
Format your log messages Evidently and persistently. Consist of context, which include timestamps, request IDs, and function names, so it’s simpler to trace issues in dispersed systems or multi-threaded environments. Structured logging (e.g., JSON logs) may make it even easier to parse and filter logs programmatically.
Through debugging, logs let you observe how variables evolve, what situations are achieved, and what branches of logic are executed—all without having halting This system. They’re Particularly precious in manufacturing environments wherever stepping via code isn’t attainable.
Additionally, use logging frameworks and applications (like Log4j, Winston, or Python’s logging module) that assistance log rotation, filtering, and integration with checking dashboards.
Finally, sensible logging is about harmony and clarity. Which has a effectively-considered-out logging approach, you can decrease the time it will require to spot concerns, get further visibility into your applications, and Enhance the Over-all maintainability and dependability of your respective code.
Imagine Like a Detective
Debugging is not only a complex endeavor—it is a form of investigation. To efficiently establish and repair bugs, developers have to tactic the procedure similar to a detective solving a mystery. This attitude will help stop working complex problems into manageable elements and comply with clues logically to uncover the basis bring about.
Get started by accumulating proof. Think about the signs and symptoms of the trouble: error messages, incorrect output, or functionality troubles. The same as a detective surveys a criminal offense scene, accumulate just as much appropriate data as you can without jumping to conclusions. Use logs, exam conditions, and person stories to piece jointly a transparent image of what’s taking place.
Subsequent, kind hypotheses. Question by yourself: What may be triggering this conduct? Have any modifications lately been produced to the codebase? Has this situation transpired prior to underneath related situations? The objective should be to slim down prospects and determine prospective culprits.
Then, check your theories systematically. Try to recreate the trouble inside a managed ecosystem. For those who suspect a particular function or part, isolate it and validate if The problem persists. Like a detective conducting interviews, question your code inquiries and Allow the outcomes guide you closer to the reality.
Spend near awareness to modest aspects. Bugs typically hide from the least predicted locations—similar to a lacking semicolon, an off-by-a single mistake, or even a race ailment. Be comprehensive and affected individual, resisting the urge to patch The problem without entirely understanding it. Short term fixes could cover the real dilemma, just for it to resurface later.
And lastly, keep notes on Whatever you tried using and discovered. Equally as detectives log their investigations, documenting your debugging course of action can conserve time for foreseeable future issues and aid Some others understand your reasoning.
By pondering similar to a detective, builders can sharpen their analytical abilities, technique complications methodically, and turn out to be simpler at uncovering concealed challenges in complicated techniques.
Produce Checks
Writing exams is one of the simplest ways to boost your debugging capabilities and Over-all enhancement efficiency. Tests not just aid catch bugs early but in addition function a security Web that offers you confidence when creating modifications in your codebase. A properly-examined application is simpler to debug since it lets you pinpoint accurately where by and when a problem takes place.
Get started with device assessments, which center on particular person features or modules. These modest, isolated assessments can speedily expose irrespective of whether a selected bit of logic is Doing work as anticipated. Each time a check fails, you instantly know where to look, noticeably lessening enough time used debugging. Device exams are Particularly useful for catching regression bugs—challenges that reappear just after Earlier getting fixed.
Future, combine integration exams and stop-to-finish checks into your workflow. These assist ensure that many areas of your application do the job jointly easily. They’re particularly handy for catching bugs that manifest in intricate methods with various parts or solutions interacting. If a little something breaks, your exams can show you which Section of the pipeline failed and underneath what circumstances.
Crafting assessments also forces you to Assume critically about your code. To check a function adequately, you will need to be familiar with its inputs, anticipated outputs, and edge conditions. This amount of being familiar with By natural means potential customers to higher code structure and less bugs.
When debugging a difficulty, writing a failing examination that reproduces the bug can be a strong starting point. Once the examination fails continuously, you are able to center on fixing the bug and enjoy your test pass when The problem is fixed. This approach makes sure that the exact same bug doesn’t return in the future.
In brief, composing checks turns debugging from the irritating guessing activity into a structured and predictable approach—encouraging you capture much more bugs, more quickly plus much more reliably.
Choose Breaks
When debugging a tricky problem, it’s straightforward to become immersed in the situation—gazing your display screen for hrs, hoping Alternative after Answer. But Just about the most underrated debugging equipment is actually stepping away. Using breaks will help you reset your head, lower annoyance, and often see the issue from the new standpoint.
If you're much too near the code for far too extensive, cognitive exhaustion sets in. You would possibly start out overlooking evident glitches or misreading code you wrote just hrs before. With this condition, your brain gets to be much less efficient at trouble-resolving. A brief walk, a coffee crack, or maybe switching to a distinct activity for ten–15 minutes can refresh your focus. Lots of builders report obtaining the root of a problem when they've taken time and energy to disconnect, letting their subconscious work during the qualifications.
Breaks also aid prevent burnout, Particularly during extended debugging periods. Sitting before a screen, mentally trapped, is not merely unproductive but also draining. Stepping absent permits you to return with renewed Power in addition to a clearer frame of mind. You may instantly see a missing semicolon, a logic flaw, or a misplaced variable that eluded you ahead of.
In the event you’re trapped, an excellent general guideline would be to set a timer—debug actively for forty five–60 minutes, then have a 5–10 moment break. Use that time to maneuver around, extend, or do something unrelated to code. It could feel counterintuitive, Specially under restricted deadlines, but it really truly causes more quickly and more practical debugging In the end.
Briefly, taking breaks will not be a sign of weak point—it’s a sensible strategy. It provides your Mind space to breathe, improves your point of view, and allows you avoid the tunnel vision That always blocks your development. Debugging is really a mental puzzle, and relaxation is part of fixing it.
Master From Each Bug
Every single bug you face is a lot more than just a temporary setback—It truly is a possibility to mature as being a developer. No matter whether it’s a syntax mistake, a logic flaw, or simply a deep architectural problem, each one can educate you something beneficial should you make time to replicate and review what went wrong.
Start by asking oneself several essential issues as soon as the bug is fixed: What prompted it? Why did it go unnoticed? Could it have been caught before with better procedures like unit testing, code critiques, or logging? The answers frequently reveal blind spots in your workflow or comprehending and assist you to Develop stronger coding habits moving ahead.
Documenting bugs will also be an outstanding practice. Continue to keep a developer journal or manage a log in which you Notice down bugs you’ve encountered, how you solved them, and Everything you learned. Over time, you’ll start to see styles—recurring difficulties or widespread blunders—that you could proactively avoid.
In workforce environments, sharing That which you've uncovered from a bug with your peers is usually In particular strong. Regardless of whether it’s through a Slack concept, a short generate-up, or A fast understanding-sharing session, encouraging Some others avoid the exact situation boosts group performance and cultivates a more powerful learning lifestyle.
Much more importantly, viewing bugs as lessons shifts your way of thinking from disappointment to curiosity. Instead of dreading bugs, you’ll start off appreciating them as important portions of your advancement journey. In fact, several of the best builders are not the ones who generate excellent code, but individuals that continually master from their blunders.
Eventually, Every bug you fix adds a different layer for your ability established. So subsequent time you squash a bug, take a instant to reflect—you’ll arrive absent a smarter, extra capable developer on account of it.
Summary
Enhancing your debugging capabilities usually takes time, apply, and endurance — though the Gustavo Woltmann AI payoff is huge. It would make you a far more efficient, assured, and able developer. Another time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.